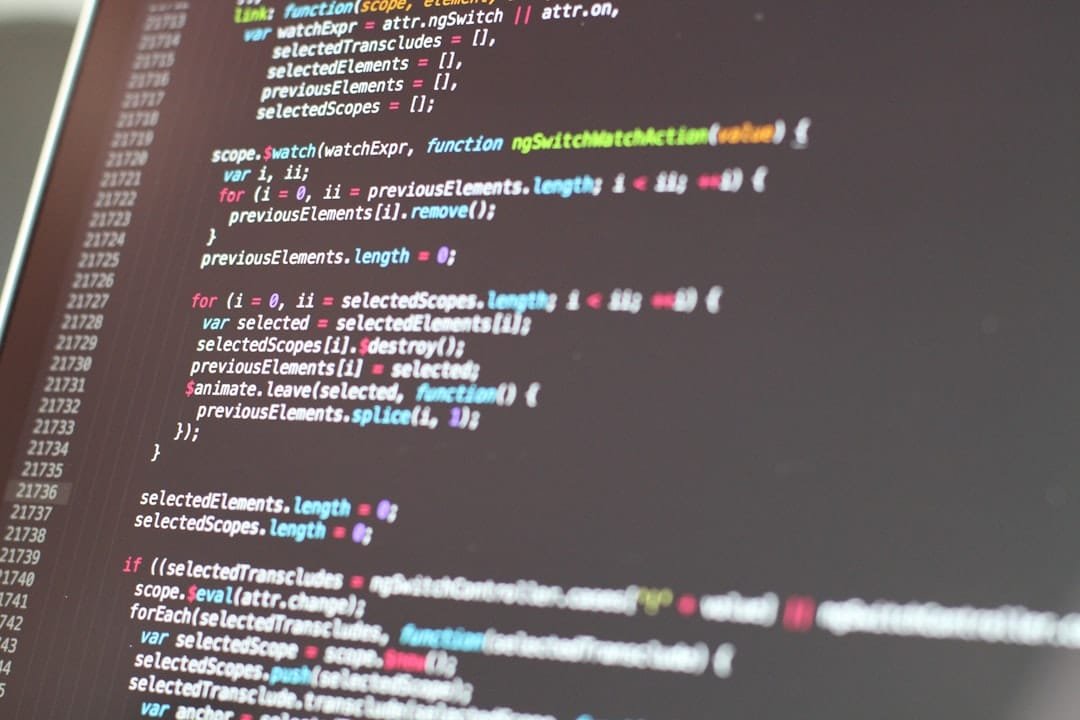
Top PHP Interview Questions: Prepare for Success
Are you gearing up for a PHP interview? Whether you’re a fresher or a seasoned developer, mastering common PHP interview questions is crucial for landing your dream job. In this simple guide, we’ll address the top PHP interview questions that recruiters frequently ask, helping you boost your confidence and ace your next interview.
Thank you for reading this post, don't forget to subscribe!The Importance of Preparing for PHP Interviews
PHP remains one of the most popular server-side programming languages, powering millions of websites and applications worldwide. As a result, the demand for skilled PHP developers continues to grow. But with great opportunity comes great competition. To stand out from the crowd, you need to be well-prepared for your interview.
Top PHP Interview Questions for Freshers
If you’re just starting your career in PHP development, you’ll likely face questions that test your fundamental knowledge of the language. Here are some key areas to focus on:
- What is PHP and what are its main features? PHP (Hypertext Preprocessor) is a server-side scripting language designed for web development. Its key features include:
- Easy to learn and use
- Cross-platform compatibility
- Support for multiple databases
- Open-source nature
- Large community support
- Extensive library support
- Explain the difference between echo and print in PHP. Both
echo
andprint
are used to output data in PHP, but they have slight differences:echo
is slightly faster and can take multiple parametersprint
can only take one argument and always returns 1
Example:
echo "Hello", " ", "World"; // Valid print "Hello World"; // Valid print "Hello", "World"; // Invalid
- What are the data types in PHP? PHP supports eight primitive data types:
- Integer
- Float (floating point numbers)
- String
- Boolean
- Array
- Object
- NULL
- Resource
Example:
$int = 42; $float = 3.14; $string = "Hello World"; $bool = true; $array = [1, 2, 3]; $object = new stdClass(); $null = NULL; $resource = fopen("example.txt", "r");
- How do you define a constant in PHP? Constants in PHP are defined using the
define()
function or theconst
keyword:define("PI", 3.14159); const MAX_VALUE = 100; echo PI; // Outputs: 3.14159 echo MAX_VALUE; // Outputs: 100
- What is the difference between
==
and===
in PHP?==
compares values but not types===
compares both values and types
Example:
$a = 5; $b = "5"; var_dump($a == $b); // Outputs: bool(true) var_dump($a === $b); // Outputs: bool(false)
- Explain the concept of variables in PHP. Variables in PHP are used to store data. They are denoted by a dollar sign followed by the variable name. PHP is a loosely typed language, which means you don’t need to declare the type of a variable explicitly.Example:
$name = "John"; $age = 30; $is_student = false; echo "Name: $name, Age: $age";
- What are the different types of arrays in PHP? PHP supports three types of arrays:
- Indexed arrays (with numeric keys)
- Associative arrays (with named keys)
- Multidimensional arrays (arrays containing other arrays)
Example:
// Indexed array $fruits = ["Apple", "Banana", "Orange"]; // Associative array $person = [ "name" => "Alice", "age" => 25, "city" => "New York" ]; // Multidimensional array $matrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ];
- How do you handle errors in PHP? PHP provides several ways to handle errors:
- Using the
try-catch
block for exception handling - Using the
error_reporting()
function to set which errors are reported - Using custom error handlers with
set_error_handler()
Example:
try { $result = 10 / 0; } catch (DivisionByZeroError $e) { echo "Error: " . $e->getMessage(); }
- Using the
- What is the purpose of the
include
andrequire
statements in PHP? Bothinclude
andrequire
are used to include and evaluate the specified file. The main difference is:- If the file is not found,
include
will only produce a warning, whilerequire
will produce a fatal error.
Example:
include 'header.php'; require 'config.php';
- If the file is not found,
- Explain the concept of sessions in PHP. Sessions in PHP are used to store user information to be used across multiple pages. They allow you to persist data for a specific user from page to page.Example:
session_start(); $_SESSION['username'] = 'JohnDoe'; echo $_SESSION['username']; // Outputs: JohnDoe
These questions form the foundation of PHP knowledge that every fresher should be familiar with. Make sure you can explain these concepts clearly and provide simple examples when possible.
PHP Interview Questions for 5 Years Experience
For developers with around 5 years of experience, interviewers will expect a deeper understanding of PHP and its ecosystem. Here are some questions you might encounter:
- Explain the concept of namespaces in PHP. Namespaces in PHP help avoid name conflicts between classes, functions, and constants. They allow you to organize code into logical groups and make it easier to reuse code across projects.Example:
namespace App\Controllers; class UserController { // Controller code here } // Using the class $controller = new \App\Controllers\UserController();
- What are traits in PHP and how are they used? Traits are a mechanism for code reuse in single inheritance languages like PHP. They allow you to reuse sets of methods freely in several independent classes living in different class hierarchies.Example:
trait Loggable { public function log($message) { echo "Logging: $message"; } } class User { use Loggable; } $user = new User(); $user->log("User created"); // Outputs: Logging: User created
- How does PHP handle exceptions? PHP uses a try-catch block for exception handling:
try { // Code that might throw an exception $result = 10 / 0; } catch (DivisionByZeroError $e) { // Handle the specific exception echo "Error: " . $e->getMessage(); } catch (Exception $e) { // Handle any other exception echo "General Error: " . $e->getMessage(); } finally { // Code that always runs, regardless of whether an exception was thrown echo "This always executes"; }
- Explain the difference between
include
andrequire
in PHP. Both are used to include external files, but:require
will produce a fatal error if the file is not foundinclude
will only produce a warning, allowing the script to continue
Example:
// If config.php doesn't exist, this will produce a fatal error require 'config.php'; // If header.php doesn't exist, this will produce a warning but continue execution include 'header.php';
- What are PHP magic methods? Give examples. Magic methods in PHP are special methods that override PHP’s default action when certain actions are performed on an object. Examples include:
class MagicExample { private $data = []; public function __construct() { echo "Object created\n"; } public function __destruct() { echo "Object destroyed\n"; } public function __get($name) { return isset($this->data[$name]) ? $this->data[$name] : null; } public function __set($name, $value) { $this->data[$name] = $value; } public function __call($name, $arguments) { echo "Calling method $name with arguments: " . implode(', ', $arguments) . "\n"; } } $obj = new MagicExample(); // Outputs: Object created $obj->name = "John"; // Uses __set echo $obj->name; // Uses __get, Outputs: John $obj->nonExistentMethod("arg1", "arg2"); // Uses __call // Outputs: Calling method nonExistentMethod with arguments: arg1, arg2
- What is autoloading in PHP and how does it work? Autoloading is a mechanism in PHP that automatically loads class files when they are needed, without explicitly including them. It’s typically used with PSR-4 autoloading standard in modern PHP applications.Example:
spl_autoload_register(function ($class_name) { include $class_name . '.php'; }); // Now you can use classes without explicitly including their files $obj = new MyClass();
- Explain the differences between abstract classes and interfaces in PHP.
- Abstract classes can have both abstract and non-abstract methods, while interfaces can only have abstract methods (prior to PHP 8.0).
- A class can implement multiple interfaces but can only extend one abstract class.
- Abstract classes can have properties, while interfaces cannot (prior to PHP 8.1).
Example:
abstract class Animal { abstract public function makeSound(); public function move() { echo "Moving..."; } } interface Swimmable { public function swim(); } class Dog extends Animal implements Swimmable { public function makeSound() { echo "Woof!"; } public function swim() { echo "Dog is swimming"; } }
- What are Closures in PHP and how are they used? Closures, also known as anonymous functions, allow the creation of functions without specifying a name. They can capture variables from the outer scope.Example:
$message = "Hello"; $greet = function($name) use ($message) { return "$message, $name!"; }; echo $greet("John"); // Outputs: Hello, John!
- Explain the concept of late static binding in PHP. Late static binding refers to the ability to reference the called class in a context of static inheritance. It’s resolved at runtime, not compile time.Example:
class A { public static function who() { echo static::class; } } class B extends A {} B::who(); // Outputs: B
- What are generators in PHP and how do they work? Generators provide an easy way to implement simple iterators without the overhead or complexity of implementing a class that implements the Iterator interface.Example:
function countTo($n) { for ($i = 1; $i <= $n; $i++) { yield $i; } } foreach (countTo(5) as $number) { echo "$number "; } // Outputs: 1 2 3 4 5
These questions delve deeper into PHP’s advanced features and object-oriented programming concepts. Be prepared to discuss real-world scenarios where you’ve applied these concepts in your projects.
PHP Interview Questions for 10 Years Experience
For senior developers with around 10 years of experience, interviewers will expect a comprehensive understanding of PHP, including its internal workings, performance optimization, and architectural decisions. Here are some advanced questions you might encounter:
- Explain the PHP execution lifecycle. The PHP execution lifecycle includes:
- Request received
- PHP initializes
- Configuration files loaded (php.ini)
- Extensions loaded
- Application code parsed and compiled to opcodes
- Execution of opcodes
- Shutdown and cleanup
- What is the Zend Engine and how does it work? The Zend Engine is the open-source interpreter for PHP. It compiles PHP code into an intermediate bytecode, which is then executed. The Zend Engine handles memory management, variable scope, and other core functionalities of PHP.
- Discuss the pros and cons of using PHP’s built-in web server for development. Pros:
- Easy to set up and use
- No configuration required
- Good for quick testing
Cons:
- Not suitable for production use
- Limited features compared to full-fledged web servers
- Single-threaded, can’t handle concurrent requests efficiently
Example usage:
php -S localhost:8000
- Explain the concept of Dependency Injection and how it’s implemented in modern PHP applications. Dependency Injection is a design pattern where objects are passed into a class instead of being created inside the class. This promotes loose coupling and easier testing.Example:
class UserService { private $database; public function __construct(Database $database) { $this->database = $database; } public function getUser($id) { return $this->database->query("SELECT * FROM users WHERE id = ?", [$id]); } } // Usage $database = new MySQLDatabase(); $userService = new UserService($database);
- What are the key differences between PHP 7 and PHP 8? Some key differences include:
- JIT compilation in PHP 8
- Union types in PHP 8
- Named arguments in PHP 8
- Attributes (metadata) in PHP 8
- Nullsafe operator in PHP 8
- Improved type system and error handling
- Explain how you would optimize a PHP application for performance. Some strategies include:
- Use OPcache to cache compiled bytecode
- Implement appropriate caching strategies (e.g., Redis, Memcached)
- Optimize database queries and indexes
- Use asynchronous processing for time-consuming tasks
- Implement CDN for static assets
- Profile code to identify bottlenecks
Example of using OPcache:
opcache_compile_file('frequently_used_script.php');
- Describe the Observer pattern and provide an example in PHP. The Observer pattern allows objects to be notified automatically of any state changes in other objects.
interface Subject { public function attach(Observer $observer); public function detach(Observer $observer); public function notify(); } interface Observer { public function update(Subject $subject); } class ConcreteSubject implements Subject { private $observers = []; private $state; public function attach(Observer $observer) { $this->observers[] = $observer; } public function detach(Observer $observer) { $key = array_search($observer, $this->observers, true); if ($key !== false) { unset($this->observers[$key]); } } public function notify() { foreach ($this->observers as $observer) { $observer->update($this); } } public function setState($state) { $this->state = $state; $this->notify(); } public function getState() { return $this->state; } } class ConcreteObserver implements Observer { public function update(Subject $subject) { echo "Observer: The subject's state has changed to: " . $subject->getState() . "\n"; } } // Usage $subject = new ConcreteSubject(); $observer1 = new ConcreteObserver(); $observer2 = new ConcreteObserver(); $subject->attach($observer1); $subject->attach($observer2); $subject->setState("New State");
- How would you implement a RESTful API in PHP without using a framework? Here’s a basic example:
<?php header("Content-Type: application/json; charset=UTF-8"); $method = $_SERVER['REQUEST_METHOD']; $request = explode('/', trim($_SERVER['PATH_INFO'],'/')); switch ($method) { case 'GET': if (isset($request[0]) && $request[0] == 'users') { if (isset($request[1])) { // Get a specific user $userId = $request[1]; echo json_encode(['user_id' => $userId, 'name' => 'John Doe']); } else { // List all users echo json_encode([['id' => 1, 'name' => 'John'], ['id' => 2, 'name' => 'Jane']]); } } break; case 'POST': if ($request[0] == 'users') { // Create a new user $input = json_decode(file_get_contents('php://input'), true); echo json_encode(['status' => 'User created', 'data' => $input]); } break; // Implement PUT, DELETE methods similarly }
- Explain the concept of Reflection in PHP and provide an example. Reflection allows code to inspect and manipulate the structure and behavior of classes, methods, and properties at runtime.
class MyClass { private $secretProperty = "secret"; public function myMethod($param1, $param2) { return $param1 . $param2; } } $reflectionClass = new ReflectionClass('MyClass'); // Get private property $property = $reflectionClass->getProperty('secretProperty'); $property->setAccessible(true); $instance = new MyClass(); echo $property->getValue($instance); // Outputs: secret // Inspect method $method = $reflectionClass->getMethod('myMethod'); print_r($method->getParameters());
- How would you implement a simple Service Container in PHP? A Service Container is used for managing class dependencies and performing dependency injection.
class ServiceContainer { private $services = []; public function register($name, $closure) { $this->services[$name] = $closure; } public function get($name) { if (!isset($this->services[$name])) { throw new Exception("Service not found: $name"); } return $this->services[$name](); } } // Usage $container = new ServiceContainer(); $container->register('database', function() { return new PDO('mysql:host=localhost;dbname=mydb', 'user', 'password'); }); $container->register('userRepository', function() use ($container) { return new UserRepository($container->get('database')); }); $userRepo = $container->get('userRepository');
These questions cover advanced topics in PHP development, including design patterns, architectural concepts, and performance optimization. Senior developers should be able to discuss these concepts in depth and provide examples from their own experience.
Top PHP Interview Questions and Answers
These questions cover a range of topics and can be asked to developers at various experience levels. They test both theoretical knowledge and practical application skills.
- What is the difference between
isset()
andempty()
in PHP?isset()
checks if a variable is set and is not NULL, whileempty()
checks if a variable is empty (equals to FALSE).$var1 = 0; $var2 = null; $var3 = []; var_dump(isset($var1)); // bool(true) var_dump(isset($var2)); // bool(false) var_dump(empty($var1)); // bool(true) var_dump(empty($var3)); // bool(true)
- Explain the difference between
GET
andPOST
methods in PHP.GET
sends data through the URL, visible in the address bar. It’s used for non-sensitive data and has a size limit.POST
sends data in the request body, not visible in the URL. It’s used for sensitive data and can handle larger amounts of data.
// Accessing GET data $id = $_GET['id']; // Accessing POST data $username = $_POST['username'];
- What are PHP Super Globals?Super Globals are predefined variables in PHP that are always accessible, regardless of scope. Examples include:
$_GET
: Contains GET data$_POST
: Contains POST data$_SESSION
: Contains session variables$_COOKIE
: Contains cookie data$_SERVER
: Contains server and execution environment information
echo $_SERVER['HTTP_USER_AGENT'];
- How do you prevent SQL injection in PHP?Use prepared statements with parameterized queries:
$stmt = $pdo->prepare("SELECT * FROM users WHERE username = :username"); $stmt->execute(['username' => $username]); $user = $stmt->fetch();
- What is the difference between
include
,require
,include_once
, andrequire_once
?include
: Includes and evaluates a file, produces a warning on failurerequire
: Same as include, but produces a fatal error on failureinclude_once
: Includes the file only if it hasn’t been included beforerequire_once
: Same as include_once, but produces a fatal error on failure
include 'config.php'; require 'functions.php'; include_once 'header.php'; require_once 'database.php';
- Explain the concept of sessions in PHP.Sessions are a way to store information across multiple pages for a single user. They use a unique session ID to identify each user.
session_start(); $_SESSION['username'] = 'John'; echo $_SESSION['username'];
- What is the purpose of the
final
keyword in PHP?Thefinal
keyword prevents child classes from overriding a method or prevents a class from being inherited.final class FinalClass { final public function finalMethod() { // This method cannot be overridden } }
- How do you handle file uploads in PHP?File uploads are handled using the
$_FILES
superglobal and themove_uploaded_file()
function.if ($_SERVER['REQUEST_METHOD'] === 'POST') { $uploadDir = '/uploads/'; $uploadFile = $uploadDir . basename($_FILES['userfile']['name']); if (move_uploaded_file($_FILES['userfile']['tmp_name'], $uploadFile)) { echo "File is valid, and was successfully uploaded."; } else { echo "Upload failed."; } }
- What is the difference between
==
and===
in PHP?==
compares values after type juggling, while===
compares both value and type.$a = 5; $b = "5"; var_dump($a == $b); // bool(true) var_dump($a === $b); // bool(false)
- Explain the concept of type hinting in PHP.Type hinting specifies the expected data type of an argument in a function declaration.
function processArray(array $arr): int { return count($arr); } $result = processArray([1, 2, 3]); // Valid $result = processArray("string"); // Fatal error
- What are Traits in PHP and how are they used?Traits are a mechanism for code reuse in single inheritance languages like PHP. They allow you to reuse sets of methods freely in several independent classes living in different class hierarchies.
trait Loggable { public function log($message) { echo "Logging: $message\n"; } } class User { use Loggable; } $user = new User(); $user->log("User created"); // Outputs: Logging: User created
- How do you implement error handling in PHP?PHP provides several ways to handle errors:
- Using try-catch blocks for exception handling
- Using the set_error_handler() function to define a custom error handler
- Using the error_reporting() function to control which errors are reported
try { $result = 10 / 0; } catch (DivisionByZeroError $e) { echo "Error: " . $e->getMessage(); } set_error_handler(function($errno, $errstr) { echo "Error [$errno]: $errstr"; }); error_reporting(E_ALL);
These questions cover a wide range of PHP concepts and are suitable for assessing candidates at various skill levels. Remember, the key to a successful interview is not just knowing the answers, but being able to explain concepts clearly and relate them to real-world scenarios.
PHP Coding Test Questions and Answers
- Write a PHP function to reverse a string without using the built-in
strrev()
function.function reverseString($str) { $length = strlen($str); $reversed = ''; for ($i = $length - 1; $i >= 0; $i--) { $reversed .= $str[$i]; } return $reversed; } // Test the function echo reverseString("Hello, World!"); // Outputs: !dlroW ,olleH
- Create a PHP script that checks if a given number is prime.
function isPrime($num) { if ($num <= 1) return false; for ($i = 2; $i <= sqrt($num); $i++) { if ($num % $i == 0) return false; } return true; } // Test the function $number = 17; echo isPrime($number) ? "$number is prime" : "$number is not prime";
- Write a PHP function to find the longest word in a string.
function findLongestWord($str) { $words = explode(' ', $str); $longestWord = ''; foreach ($words as $word) { if (strlen($word) > strlen($longestWord)) { $longestWord = $word; } } return $longestWord; } // Test the function $sentence = "The quick brown fox jumps over the lazy dog"; echo findLongestWord($sentence); // Outputs: quick
- Implement a simple calculator class in PHP that can perform basic arithmetic operations.
class Calculator { public function add($a, $b) { return $a + $b; } public function subtract($a, $b) { return $a - $b; } public function multiply($a, $b) { return $a * $b; } public function divide($a, $b) { if ($b == 0) { throw new Exception("Division by zero"); } return $a / $b; } } // Test the calculator $calc = new Calculator(); echo $calc->add(5, 3) . "\n"; // Outputs: 8 echo $calc->subtract(10, 4) . "\n"; // Outputs: 6 echo $calc->multiply(2, 6) . "\n"; // Outputs: 12 echo $calc->divide(15, 3) . "\n"; // Outputs: 5
- Write a PHP function to remove duplicate values from an array.
function removeDuplicates($arr) { return array_values(array_unique($arr)); } // Test the function $numbers = [1, 2, 2, 3, 4, 4, 5]; print_r(removeDuplicates($numbers)); // Outputs: Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
- Create a PHP class for a basic blog post with properties and methods.
class BlogPost { private $title; private $content; private $author; private $publishDate; public function __construct($title, $content, $author) { $this->title = $title; $this->content = $content; $this->author = $author; $this->publishDate = new DateTime(); } public function getTitle() { return $this->title; } public function getExcerpt($length = 50) { return substr($this->content, 0, $length) . '...'; } public function getAuthor() { return $this->author; } public function getPublishDate($format = 'Y-m-d H:i:s') { return $this->publishDate->format($format); } } // Test the class $post = new BlogPost("PHP Tips", "Here are some useful PHP tips...", "John Doe"); echo $post->getTitle() . "\n"; echo $post->getExcerpt() . "\n"; echo $post->getAuthor() . "\n"; echo $post->getPublishDate();
- Implement a function to check if a string is a palindrome.
function isPalindrome($str) { $str = strtolower(preg_replace('/[^a-zA-Z0-9]/', '', $str)); return $str === strrev($str); } // Test the function echo isPalindrome("A man, a plan, a canal: Panama") ? "Palindrome" : "Not a palindrome"; // Outputs: Palindrome
- Write a PHP function to generate a random password of a specified length.
function generatePassword($length = 8) { $chars = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!@#$%^&*()_+'; $password = ''; for ($i = 0; $i < $length; $i++) { $password .= $chars[rand(0, strlen($chars) - 1)]; } return $password; } // Test the function echo generatePassword(12); // Outputs a random 12-character password
- Implement a simple caching mechanism using PHP files.
class FileCache { private $cacheDir; private $cacheTime; public function __construct($cacheDir = 'cache', $cacheTime = 3600) { $this->cacheDir = $cacheDir; $this->cacheTime = $cacheTime; if (!is_dir($this->cacheDir)) { mkdir($this->cacheDir, 0755, true); } } public function set($key, $data) { $filename = $this->cacheDir . '/' . md5($key) . '.cache'; $content = serialize(['time' => time(), 'data' => $data]); file_put_contents($filename, $content); } public function get($key) { $filename = $this->cacheDir . '/' . md5($key) . '.cache'; if (file_exists($filename)) { $content = unserialize(file_get_contents($filename)); if (time() - $content['time'] < $this->cacheTime) { return $content['data']; } } return false; } } // Test the cache $cache = new FileCache(); $cache->set('user', ['name' => 'John', 'age' => 30]); $user = $cache->get('user'); print_r($user);
- Create a PHP function to flatten a multidimensional array.
function flattenArray($array) { $result = []; array_walk_recursive($array, function($value) use (&$result) { $result[] = $value; }); return $result; } // Test the function $nestedArray = [1, [2, 3, [4, 5]], 6, [7, 8]]; print_r(flattenArray($nestedArray)); // Outputs: Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 [5] => 6 [6] => 7 [7] => 8 )
These coding test questions cover a range of PHP programming concepts and problem-solving skills. They test a candidate’s ability to write clean, efficient code and implement common programming patterns.
Beginner Laravel Interview Questions
What is Laravel, and why is it popular among PHP developers?
Laravel is a free, open-source PHP web application framework created by Taylor Otwell. It’s popular because it follows the MVC (Model-View-Controller) architectural pattern, provides elegant syntax, and offers many built-in features that simplify common web development tasks.
How do you install Laravel?
You can install Laravel using Composer, PHP’s package manager, with the following command:
composer create-project --prefer-dist laravel/laravel project-name
What is Artisan in Laravel?
Artisan is the command-line interface included with Laravel. It provides a number of helpful commands for use while developing your application. To see a list of available Artisan commands, you can use the php artisan list
command.
How do you define a route in Laravel?
Routes are defined in the routes/web.php
file for web routes and routes/api.php
for API routes. Here’s an example of a basic route:
Route::get('/hello', function () {
return 'Hello, World!';
});
What are migrations in Laravel?
Migrations are like version control for your database. They allow you to define and share your application’s database schema. You can create a migration using the Artisan command:
php artisan make:migration create_users_table
How do you create a new controller in Laravel?
You can create a new controller using the Artisan command:
php artisan make:controller UserController
What is Blade templating in Laravel?
Blade is the simple, yet powerful templating engine provided with Laravel. It doesn’t restrict you from using plain PHP code in your views. All Blade views are compiled into plain PHP code and cached until they are modified.
How do you access configuration values in Laravel?
You can access configuration values using the config()
helper function:
$value = config('app.timezone');
What is the purpose of the .env file in Laravel?
The .env
file is used to store configuration values that may vary between deployment environments (local, staging, production). It keeps sensitive information out of your code.
How do you create a new model in Laravel?
You can create a new model using the Artisan command:
php artisan make:model User
These questions cover the basics of Laravel and are suitable for beginners.
Mid-Level Laravel Interview Questions
Explain the Laravel service container and dependency injection.
The service container is a powerful tool for managing class dependencies and performing dependency injection. It can automatically resolve dependencies in controllers, event listeners, and other parts of your application. Example:
public function __construct(UserRepository $users)
{
$this->users = $users;
}
What are Laravel facades and how do they work?
Facades provide a static interface to classes that are available in the application’s service container. They serve as a static proxy to underlying classes, offering the benefit of a terse, expressive syntax while maintaining testability and flexibility. Example:
use Illuminate\Support\Facades\Cache;
Cache::get('key');
Describe Laravel’s Eloquent ORM and its advantages.
Eloquent is Laravel’s built-in ORM (Object-Relational Mapping). It provides an ActiveRecord implementation for working with your database. Each database table has a corresponding “Model” that is used to interact with that table. Advantages include:
- Intuitive query building
- Relationships management
- Data manipulation using PHP objects
- How do you handle authentication in Laravel?
Laravel makes implementing authentication very simple. You can use the auth
middleware, the Auth
facade, or the auth()
helper function. Laravel also provides scaffolding for authentication with the laravel/ui
package or Laravel Jetstream.
Explain Laravel’s validation process.
Laravel provides several approaches to validate your application’s incoming data:
- Controller validation
- Form request validation
- Manually creating validators
Example of validation in a controller:
$validatedData = $request->validate([
'title' => 'required|unique:posts|max:255',
'body' => 'required',
]);
What are Laravel jobs and how do you use them?
Jobs are used for queueing tasks that are time-consuming or need to be run asynchronously. You can create a job class and dispatch it:
dispatch(new ProcessPodcast($podcast));
How do you implement caching in Laravel?
Laravel supports various cache drivers (file, database, Memcached, Redis). You can use the Cache
facade or cache()
helper function:
Cache::put('key', 'value', $seconds);
$value = Cache::get('key');
Explain Laravel’s event broadcasting system.
Event broadcasting allows you to broadcast your server-side Laravel events to your client-side JavaScript application. It’s useful for real-time applications. You can use Laravel Echo on the client-side to listen for these events.
How do you create and use middleware in Laravel?
Middleware provide a convenient mechanism for filtering HTTP requests entering your application. You can create middleware using Artisan:
php artisan make:middleware CheckAge
Then register it in app/Http/Kernel.php
and use it in routes:
Route::get('admin/profile', function () {
//
})->middleware('auth');
What are Laravel’s queues and how do they work?
Queues allow you to defer the processing of a time-consuming task, such as sending an email, which drastically speeds up web requests to your application. Laravel supports various queue backends like Amazon SQS, Redis, and database.
These mid-level questions delve deeper into Laravel’s architecture and advanced features. They test a developer’s ability to leverage Laravel’s powerful tools to build robust applications.
Senior Laravel Developer Interview Questions
Explain Laravel’s service provider concept and how you would create a custom service provider.
Service providers are the central place of all Laravel application bootstrapping. They are responsible for binding things into Laravel’s service container and informing Laravel where to load package resources such as views, configuration, and localization files.
To create a custom service provider:
php artisan make:provider CustomServiceProvider
class CustomServiceProvider extends ServiceProvider
{
public function register()
{
$this->app->singleton('custom-service', function ($app) {
return new CustomService();
});
}
public function boot()
{
//
}
}
- How would you optimize a Laravel application for better performance?
- Use eager loading to reduce N+1 query problems
- Implement caching strategies (Redis, Memcached)
- Use query caching for frequently accessed data
- Optimize database indexes
- Use Laravel’s route caching in production
- Implement queue workers for time-consuming tasks
- Use Laravel Mix for asset compilation and versioning
- Implement API rate limiting
- Use Laravel Telescope for debugging and monitoring
- Explain Laravel’s package development process and how you would create a custom package.
Creating a custom Laravel package involves:
- Set up package structure
- Create a service provider
- Define package configuration
- Write package code
- Create package routes, views, and migrations
- Write tests for your package
- Create a composer.json file
- Publish your package on Packagist
Example structure:
my-package/
src/
MyPackageServiceProvider.php
config/
my-package.php
resources/
views/
routes/
web.php
tests/
composer.json
How does Laravel handle database transactions, and when would you use them?
Laravel supports database transactions, which allow you to wrap a set of operations in a database transaction to ensure they are executed as a single unit. You can use them like this:
DB::transaction(function () {
DB::table('users')->update(['votes' => 1]);
DB::table('posts')->delete();
});
Use transactions when you have multiple database operations that need to succeed or fail as a group.
Explain Laravel’s event sourcing capabilities and when you might use this pattern.
Event sourcing is a pattern where the state of your application is determined by a sequence of events rather than just the current state. Laravel provides event sourcing capabilities through packages like Spatie’s Laravel Event Sourcing.
You might use event sourcing when:
- You need a complete audit trail of all changes
- You want to be able to reconstruct past states of your application
- You’re building a system with complex business rules that change over time
- How would you implement a multi-tenancy architecture in Laravel?
There are several approaches to multi-tenancy in Laravel:
- Separate databases: Each tenant has their own database.
- Separate schemas: Tenants share a database but have separate schemas.
- Discriminator column: All tenants share tables, but rows are filtered by a tenant_id column.
You could implement this using middleware to determine the current tenant, and then use Laravel’s multiple database connections or query scopes to filter data appropriately.
Explain Laravel’s approach to testing and how you would set up a comprehensive testing strategy for a large Laravel application.
Laravel provides a rich testing suite built on PHPUnit. A comprehensive testing strategy might include:
- Unit tests for individual classes and methods
- Feature tests for testing entire features or endpoints
- Browser tests using Laravel Dusk for frontend testing
- API tests for testing API endpoints
- Database tests for testing database operations
- Mocking external services and dependencies
- Continuous Integration setup (e.g., with GitHub Actions or GitLab CI)
- Code coverage reports
- How would you implement a robust error handling and logging system in a Laravel application?
- Use Laravel’s built-in exception handler in
app/Exceptions/Handler.php
- Implement custom exception classes for specific error types
- Use Laravel’s logging system, possibly with a service like Bugsnag or Sentry for production environments
- Implement proper HTTP status codes for API responses
- Use try-catch blocks for anticipated exceptions
- Implement a consistent error response format for APIs
- Explain how you would design and implement a scalable real-time notification system using Laravel and WebSockets.
- Use Laravel’s event broadcasting system
- Implement Laravel Echo server or a service like Pusher
- Use Laravel’s notification system for creating notifications
- Implement a queue system for processing notifications
- Use Redis for managing presence channels and temporary data storage
- Implement proper authentication for WebSocket connections
- Consider horizontal scaling for WebSocket servers in high-load situations
- How would you approach building a large-scale, modular Laravel application? Discuss architectural decisions and best practices.
- Use Laravel’s built-in module system or a package like nWidart/laravel-modules
- Implement Domain-Driven Design principles
- Use SOLID principles in your code design
- Implement a service layer between controllers and models
- Use dependency injection and the service container extensively
- Implement a consistent coding standard (PSR-12)
- Use Laravel’s built-in features like policies and form requests for authorization and validation
- Implement a robust testing strategy
- Use Laravel Horizon for managing and monitoring queue workers
- Implement proper caching strategies
- Use Laravel Telescope in development for debugging and performance monitoring
These senior-level questions test a deep understanding of Laravel’s architecture, advanced features, and best practices for building large-scale applications. They also cover important aspects of system design, performance optimization, and architectural decision-making that a senior Laravel developer should be familiar with.
PHP Best Practices, Common Pitfalls, and Advanced Topics
- Security Best Practicesa) Always validate and sanitize user input:
$email = filter_var($_POST['email'], FILTER_SANITIZE_EMAIL); if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { // Invalid email }
b) Use prepared statements to prevent SQL injection:
$stmt = $pdo->prepare("SELECT * FROM users WHERE username = :username"); $stmt->execute(['username' => $username]);
c) Implement CSRF protection:
session_start(); if (!isset($_SESSION['csrf_token'])) { $_SESSION['csrf_token'] = bin2hex(random_bytes(32)); }
- Performance Optimizationa) Use opcode caching (like OPcache):
opcache_compile_file('frequently_used_script.php');
b) Implement application-level caching:
$cache = new Memcached(); $cache->add('key', 'value', 3600); // Cache for 1 hour
c) Optimize database queries:
// Use LIMIT for pagination $stmt = $pdo->prepare("SELECT * FROM posts LIMIT :offset, :limit"); $stmt->execute(['offset' => 0, 'limit' => 10]);
- Common Pitfallsa) Forgetting to escape output:
// Bad echo $_GET['user_input']; // Good echo htmlspecialchars($_GET['user_input'], ENT_QUOTES, 'UTF-8');
b) Not setting the correct content type:
header('Content-Type: application/json'); echo json_encode($data);
c) Using
==
instead of===
for comparisons:// This condition is true if ('0' == false) { echo "This will be printed!"; } // This condition is false if ('0' === false) { echo "This won't be printed."; }
- Advanced Topicsa) Generators for memory-efficient iteration:
function xrange($start, $end, $step = 1) { for ($i = $start; $i <= $end; $i += $step) { yield $i; } } foreach (xrange(1, 1000000) as $num) { echo $num . "\n"; }
b) Anonymous classes (PHP 7+):
$util = new class { public function doSomething() { return "Done!"; } }; echo $util->doSomething(); // Outputs: Done!
c) Typed properties (PHP 7.4+):
class User { public int $id; public string $name; public ?string $email; }
- Design Patterns in PHPa) Singleton Pattern:
class Database { private static $instance = null; private function __construct() {} public static function getInstance() { if (self::$instance === null) { self::$instance = new self(); } return self::$instance; } }
b) Factory Pattern:
interface Animal { public function makeSound(); } class Dog implements Animal { public function makeSound() { return "Woof!"; } } class Cat implements Animal { public function makeSound() { return "Meow!"; } } class AnimalFactory { public static function createAnimal($type) { switch ($type) { case 'dog': return new Dog(); case 'cat': return new Cat(); default: throw new Exception("Invalid animal type"); } } } $dog = AnimalFactory::createAnimal('dog'); echo $dog->makeSound(); // Outputs: Woof!
- Handling Asynchronous OperationsWhile PHP is primarily synchronous, you can use libraries like ReactPHP or Amp for asynchronous programming:
// Using ReactPHP $loop = React\EventLoop\Factory::create(); $loop->addTimer(1.0, function () { echo "Hello after 1 second\n"; }); $loop->addPeriodicTimer(0.5, function () { echo "Tick\n"; }); $loop->run();
- Working with Composer and AutoloadingComposer is the de facto package manager for PHP. Here’s how to use it for autoloading:
{ "autoload": { "psr-4": { "MyApp\\": "src/" } } }
Then in your PHP file:
require 'vendor/autoload.php'; use MyApp\SomeClass; $obj = new SomeClass();
- Error Handling and LoggingUse exceptions for error handling and a logging library like Monolog for robust logging:
use Monolog\Logger; use Monolog\Handler\StreamHandler; $log = new Logger('name'); $log->pushHandler(new StreamHandler('path/to/your.log', Logger::WARNING)); try { // Some code that might throw an exception throw new Exception("This is an error"); } catch (Exception $e) { $log->error($e->getMessage()); }
These advanced topics, best practices, and common pitfalls are crucial for experienced PHP developers to understand and implement in their projects. They contribute to creating more secure, efficient, and maintainable PHP applications.