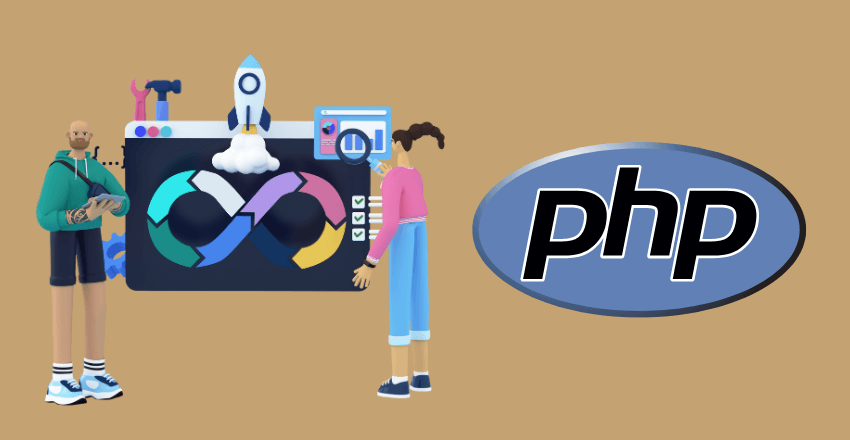
Best PHP developer hiring strategies for complex enterprise web application projects
When companies need to build complicated websites and apps, finding the right PHP developer becomes super important. Think of it like picking the best player for a championship team – you want someone who can handle tough challenges and create winning solutions.
Thank you for reading this post, don't forget to subscribe!Key Things to Look for When Hiring a PHP Developer
Strong Technical Skills
Advanced PHP Frameworks: Your Developer’s Power Tools
Think of PHP frameworks like a professional carpenter’s specialized toolset. Just as a master carpenter uses precise, purpose-built tools, top PHP developers leverage frameworks to work more efficiently. Laravel and Symfony aren’t just random tools – they’re sophisticated platforms that help developers build complex web applications faster and more securely.
Laravel: The Swiss Army Knife of Web Development
Laravel, for instance, is like a Swiss Army knife for web development. It provides built-in tools for:
- Authentication systems
- Database migrations
- Robust security features
- Efficient routing
- Elegant syntax that makes complex code more readable
Symfony: The Modular Construction Set
Symfony offers similar power, with a more modular approach. It’s like a professional-grade construction set where developers can pick and choose components exactly matching their project’s needs.
Database Design: The Foundation of Great Web Applications
Imagine a database as the nervous system of a web application. A skilled PHP developer doesn’t just store data – they design intelligent, efficient database structures. This means:
- Creating relationships between different data tables
- Optimizing query performance
- Preventing data redundancy
- Ensuring data integrity and consistency
For enterprise applications, database design isn’t just technical – it’s strategic. A great developer understands how database architecture impacts business operations, scalability, and future growth.
Web Security Protocols: Building Digital Fortresses
Web security isn’t an afterthought – it’s a critical requirement. Top PHP developers think like ethical hackers, anticipating potential vulnerabilities. They implement protection against:
- SQL injection attacks
- Cross-site scripting (XSS)
- Authentication bypass attempts
- Data encryption
- Secure user authentication mechanisms
Writing Clean, Efficient Code
Clean code is like a well-organized workspace. It’s not just about making things work – it’s about making them work beautifully. This means:
- Following consistent coding standards
- Writing comments that explain complex logic
- Creating modular, reusable code segments
- Minimizing redundant operations
- Optimizing performance through intelligent algorithms
A developer’s code should be readable not just by machines, but by other human developers. It should tell a story, with each function and class serving a clear, specific purpose.
Practical Example
Let’s look at a small code snippet demonstrating clean PHP practices:
// Validate and sanitize user input
function validateUserInput(string $input): string {
// Remove potentially harmful characters
$cleanInput = htmlspecialchars(trim($input));
// Additional validation can be added here
return $cleanInput;
}
// Example usage in a user registration process
$username = validateUserInput($_POST[‘username’]);
This short function demonstrates multiple best practices:
- Input sanitization
- Type hinting
- Clear, descriptive function name
- Single responsibility principle
Mental Exercise for Understanding
Consider asking your potential PHP developer: “Can you walk me through how you would design a secure user authentication system from scratch?” Their answer will reveal their depth of technical understanding, problem-solving approach, and awareness of security complexities.
Experience matters a lot
Picture This: You’re Building a Massive Treehouse Would you hire someone who’s only read about treehouses, or someone who’s already constructed complex, multi-level treehouses before? The same logic applies to PHP developers.
Why Experience Matters
Imagine you’re running a marathon. Book knowledge helps, but nothing prepares you like actual running experience. Real-world project experience teaches developers lessons no classroom ever could.
Breaking Down Project Experience
Large, Complex Web Projects: The Real Proving Ground
When developers work on big projects, they learn critical skills:
- Handling massive amounts of data
- Managing multiple team members
- Juggling competing technical requirements
- Understanding how different system components interact
A developer who’s built a complex e-commerce platform, for example, knows how to:
- Create scalable user authentication systems
- Design efficient payment processing workflows
- Manage high-traffic database interactions
- Balance performance with security needs
Industry-Specific Application Expertise
Not all web applications are created equal. A PHP developer who’s built applications in your industry brings invaluable insights. They understand:
- Specific industry challenges
- Unique technical requirements
- Common workflow bottlenecks
- Regulatory compliance needs
Example: A developer who’s created healthcare management systems will understand HIPAA compliance, data security, and patient information management far better than a generalist.
Technical Problem-Solving: The Hidden Superpower
Great developers don’t just write code—they solve puzzles. Look for stories about how they’ve tackled challenging technical problems. These stories reveal:
- Creative thinking abilities
- Persistence under pressure
- Technical depth and adaptability
Example
Let’s say a developer tells you about a time they:
- Reduced a website’s loading time from 8 seconds to 1 second
- Fixed a complex caching issue affecting thousands of users
- Integrated multiple third-party APIs with zero downtime
These stories demonstrate not just technical skill, but strategic thinking and real-world impact.
Interview Techniques to Uncover Experience
When interviewing, ask developers to walk you through:
- Their most challenging project
- A time they saved a project from potential failure
- How they collaborated with non-technical team members
- Specific technical challenges they’ve overcome
Red Flags to Watch Out For
Be cautious of developers who:
- Only discuss theoretical scenarios
- Can’t provide concrete project examples
- Seem uncomfortable explaining technical decisions
- Lack specifics about their contributions
Mental Exercise: Experience Assessment
Try this interview technique: Ask the developer to draw a system architecture diagram of their most complex previous project. Watch how they:
- Explain interconnected components
- Describe data flow
- Highlight potential bottlenecks
- Communicate technical concepts
Skill vs. Experience: Finding the Right Balance
Remember: Experience doesn’t always mean age or years worked. Look for:
- Quality of projects over quantity
- Depth of problem-solving
- Continuous learning attitude
- Demonstrable impact on previous projects
Tip: Code Repositories Matter
Check the developer’s GitHub or similar platforms. Look for:
- Consistent contribution patterns
- Open-source project involvement
- Quality of code comments
- Diversity of projects
Problem-Solving Abilities
Let’s break down what makes a PHP developer truly great at solving complex problems. It’s not just about writing code it’s about being a tech detective who can crack tough challenges.
Asking Smart Questions: The Investigation Begins
Think of a great problem-solver like a detective investigating a mystery. They don’t just jump to conclusions. They dig deep and ask questions that reveal the real story.
What Smart Questions Look Like:
- “What specific business challenge are we trying to solve?”
- “How will this solution impact our users?”
- “What are our must-have features versus nice-to-have features?”
- “What constraints do we need to work around?”
Example Scenario:
A company wants a new customer management system. A mediocre developer might say, “I’ll build you a system.” A great developer asks:
- “How many customers do you expect to manage?”
- “What unique workflows does your business need?”
- “What existing systems must this integrate with?”
- “What’s your budget for maintenance and future upgrades?”
These questions reveal crucial details that transform a generic solution into a perfectly tailored system.
Creative Problem-Solving: Thinking Outside the Code Box
Creative problem-solving isn’t about being fancy—it’s about finding unexpected paths to solutions.
Imagine you’re trying to cross a river. A basic thinker builds a bridge. A creative problem-solver might:
- Find a better river crossing point
- Design a lightweight boat
- Create a tunnel underneath
- Develop a completely new transportation method
In PHP development, creativity looks like:
- Finding performance improvements
- Designing elegant workarounds for technical limitations
- Combining multiple tools in unexpected ways
- Simplifying complex processes
Creative Solving Example:
A developer discovers a client’s website crashes during high-traffic events. Instead of just adding more servers, they:
- Optimize database queries
- Implement intelligent caching mechanisms
- Create a scalable load-balancing strategy
- Reduce unnecessary data processing
Explaining Technical Concepts
Great developers don’t hide behind technical jargon. They explain complex ideas so your grandma could understand them.
Communication Techniques:
- Use real-world analogies
- Avoid technical buzzwords
- Break down complex ideas into simple steps
- Draw visual diagrams
- Check for understanding
Example Explanation:
Instead of saying: “We’ll implement a microservices architecture with containerized deployment using Kubernetes,” say: “Imagine your website is like a big LEGO set. Instead of one massive block, we’ll create smaller, specialized blocks that can be easily replaced or upgraded. This means if one part breaks, the entire system doesn’t crash.”
Interview Technique: The Explanation Test
When interviewing, ask developers to explain a complex technical concept. Watch for:
- Clear, simple language
- Patience in explaining
- Ability to read your understanding
- Enthusiasm for the topic
Red Flags in Technical Communication:
Be cautious of developers who:
- Use excessive technical jargon
- Become defensive when asked to clarify
- Talk down to non-technical team members
- Can’t simplify complex concepts
Communication Skills Technical talent isn’t enough
- Explain complex ideas clearly
- Work well with different team members
- Update project stakeholders regularly
- Understand business requirements
Continuous Learning Technology changes fast
- Stay updated on new programming techniques
- Learn new tools and frameworks quickly
- Attend workshops and tech conferences
- Read industry blogs and publications
Portfolio and References Always check a developer’s past work
- Examples of completed web applications
- Links to GitHub or professional coding profiles
- References from previous employers
- Detailed explanation of their specific contributions
Cultural Fit Technical skills are crucial, but team chemistry matters too
- Match your company’s work style
- Show enthusiasm for your project
- Demonstrate reliability and professionalism
- Can collaborate effectively with your existing team
Interview and Assessment Tips
When interviewing PHP developers, try these strategies:
- Give them a real-world coding challenge
- Ask about past project difficulties and solutions
- Discuss their approach to handling complex technical problems
- Check their passion for web development
Salary and Compensation
Expect to pay competitive rates for top talent. PHP developers with enterprise experience typically earn between $80,000 and $130,000 annually, depending on skills and location.
Finding the right PHP developer takes time and careful screening. Don’t rush the process. Take your time, ask great questions, and look for someone who brings both technical excellence and creative problem-solving to your team.